Create a React app
Contents
In this chapter you will create a React application with TypeScript support.
Create React app
With the sandbox running, open a new terminal tab/window and run the following command:
npm create vite@6.1.1 react-enonic -- --template react-ts
This will create a React app and place it in a folder called react-enonic/
.
Install dependencies
With the basic project files in place, you must add some handy npm modules as well:
cd react-enonic
npm install --save react-router-dom sass @enonic/react-components@5.0.1 @enonic/js-utils
Configure
The React application now needs to know the location of the Enonic API, as we’ll need it for fetching data later.
Open an IDE of your choice, and create an .env
file in the root of your project, containing a single line:
VITE_GUILLOTINE_URL=http://localhost:8080/site/intro/master
which is the URL you used to reach Query Playground when setting up Enonic, with appended /master
.
/master is added to the URL because we want to access the master branch (published content). |
Wonder why it is called Guillotine? Guillotine is simply the name for Enonic’s headless GraphQL API.
Start
Run this command from inside your project’s folder:
npm run dev -- --port 3000
This will launch the app on port 3000 in your default browser.
Visit http://localhost:3000 directly if it does not launch automatically |
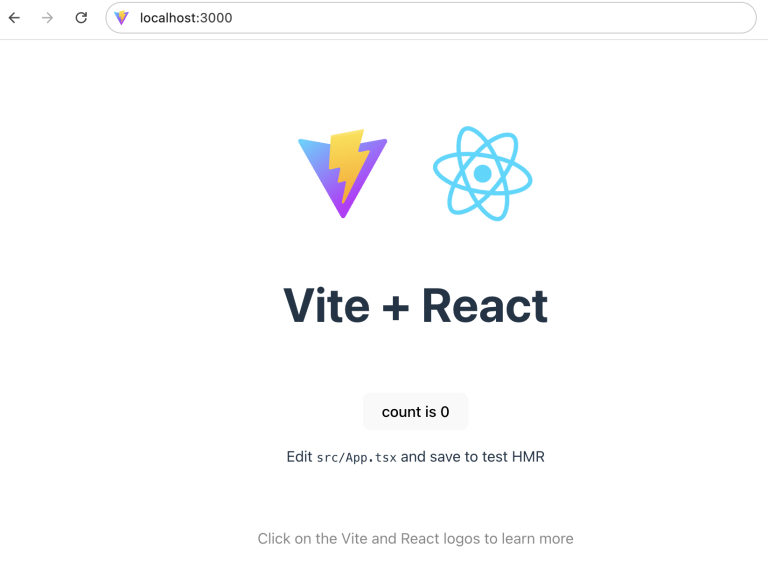
React router
Although beautiful, the app doesn’t do much yet and just shows the Vite+React splash screen. Let’s begin by adding a router and some custom styling:
-
Add a file called
App.sass
to the/src
folder. We’ll use it instead of the built-inApp.css
.src/App.sassbody background-color: #fff color: #595959 // 7:1 AAA #595959 on #fff padding: 0 margin: 0 font-family: roboto, arial, serif width: 100% box-sizing: border-box .back-link padding: 30px
-
Then update
App.tsx
file with the following code:src/App.tsximport './App.sass'; import { Route, BrowserRouter as Router, Routes, } from 'react-router-dom'; export default function App() { return ( <Router> <Routes> <Route path='*' element={<p>Hello router!</p>}/> </Routes> </Router> ) }
-
Your browser should now display the text
Hello router!
.
Next step
In the following chapter you’ll create a proper React component - the Person list.