The Rich text component
Contents
In this chapter we’ll look at how you can take control over the Enonic’s rich text component.
Background
The RichText component is able to output HTML, and comes with basic handling of links and images.
URLs generated by default may not suit your use-case, which is why you can implement your own Image
and Link
components, and inject them into the RichText component.
Links
The Link
component is used by RichText
to correctly resolve and render links inside an HTML content.
The default implementation generates links based on the href
included in the GraphQL query. To see this, visit the Lea Seydoux page and click the Daniel Craig link inside her biography:
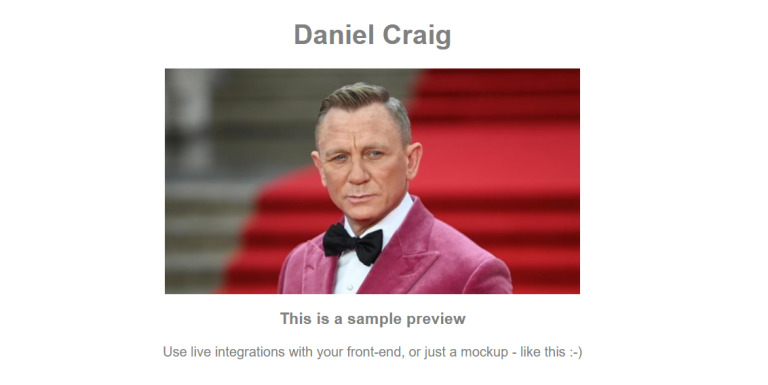
What we get here is a default preview generated by Enonic XP and not by our React app.
Our goal is to change internal person links to be in the format /p/:name/:personId
- so they will be rendered by the React application instead.
Custom link component
Add the following code to your project:
import type {LinkComponent} from '@enonic/react-components';
export const Link: LinkComponent = ({
children,
content,
media,
href,
target,
title,
uri,
...rest
}) => {
let appHref = '';
if (content && content.type?.endsWith(':person')) {
appHref = `/p/${content?._name}/${content?._id}`;
} else if (media?.content) {
appHref = href;
} else {
return <>{children}</>
}
return <a href={appHref} target={target} title={title}>{children}</a>;
}
What does it do?
-
For the
person
content type, we construct URLs in this format/p/:name/:personId
. -
For media content, we keep the
href
attribute, because we want media to be served directly from Enonic. -
For all other links, we remove the links and render text because our app can not handle it.
Finally, we must also ensure that we inject the new component into the RichText
component:
import {Link} from './Link';
// ...
<RichText
// ...
Link={Link}
// ...
/>
If you go to the Lea Seydoux page and click the Daniel Craig link again, you should see that the Daniel Craig’s page is now rendered by our React app:
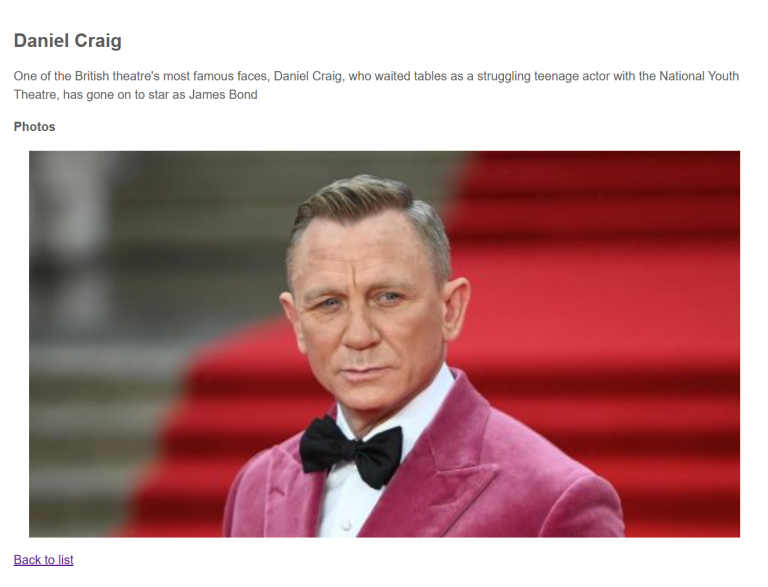
Images
Similarly, you can implement your own Image
component in order to take more control over how images are rendered:
import type {ImageComponent} from '@enonic/react-components';
export const Image: ImageComponent = ({
alt,
image,
imageStyle,
sizes,
src,
srcSet,
style,
...rest
}) => {
return <img
alt={alt}
sizes={sizes}
src={src}
srcSet={srcSet}
style={style}
/>;
}
The above simply keeps the standard values, but can be considered a starting point.
Remember to add your image component to the RichText
component like this:
import {Image} from './Image';
// ...
<RichText
...
Image={Image}
...
/>